- WindowsAzure.Storage
- Microsoft.WindowsAzure.ConfigurationManager
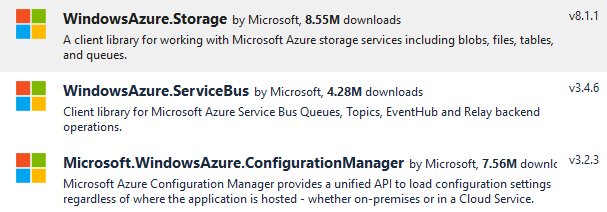
here is the extract from packages.config
<packages>
<package id="Microsoft.WindowsAzure.ConfigurationManager"
version="3.2.3" targetFramework="net40" />
<package id="WindowsAzure.Storage"
version="8.1.1" targetFramework="net40" />
</packages>
web.config
<add key="storage:account:name" value="d8view"/> <add key="storage:account:key" value="7o1-----A=="/><add key="storage:account:connection" value="DefaultEndpointsProtocol=https;AccountName=d8view;AccountKey=7o1-----==" /><add key="storage:account:PDFContainer" value="patients"/>
using Microsoft.WindowsAzure.Storage; using Microsoft.WindowsAzure.Storage.Blob;declare the CloudBlobClient!
CloudBlobClient blobClient; public constructor() { // Retrieve storage account from connection string. CloudStorageAccount storageAccount = CloudStorageAccount.Parse( ConfigurationManager.AppSettings["storage:account:connection"]); // Create the blob client. blobClient = storageAccount.CreateCloudBlobClient(); }
Retrive the blob with policy url
public string GetAzureBlobReference(string strContainer, string prefix) { // Retrieve reference to a previously created container. CloudBlobContainer container = blobClient.GetContainerReference(strContainer); var readPolicy = new SharedAccessBlobPolicy() { Permissions = SharedAccessBlobPermissions.Read, SharedAccessExpiryTime = DateTime.UtcNow + TimeSpan.FromMinutes(5) }; var docref = container.GetBlobReference(prefix); return docref.Uri.AbsoluteUri + docref.GetSharedAccessSignature(readPolicy) }; }
download as byte[]
//download document templete from Azurebolb
public byte[] DownloadTemplate(string templateName)
{
byte[] templateInByteArray;
try
{
//string containerName = "template";
string fileName = templateName;
var accountName = ConfigurationManager.AppSettings["storage:account:name"];
var accountKey = ConfigurationManager.AppSettings["storage:account:key"];
var containerName = ConfigurationManager.AppSettings["storage:WordTemplate:Location"];
var storageAccount = new CloudStorageAccount(new StorageCredentials(accountName, accountKey), true);
CloudBlobClient blobClient = storageAccount.CreateCloudBlobClient();
// Retrieve reference to a previously created container.
CloudBlobContainer container = blobClient.GetContainerReference(containerName);
// Retrieve reference to a blob
CloudBlockBlob blockBlob = container.GetBlockBlobReference(fileName);
using (MemoryStream ms = new MemoryStream())
{
blockBlob.DownloadToStream(ms);
templateInByteArray = ms.ToArray();
}
}
return templateInByteArray;
}
upload blob
//update generated pdf Azurebolb
public bool UploadPDFToAzureBlob(byte[] pdf, string fileName)
{
try
{
//string containerName = "generatedpdf";
var accountName = ConfigurationManager.AppSettings["storage:account:name"];
var accountKey = ConfigurationManager.AppSettings["storage:account:key"];
var containerName = ConfigurationManager.AppSettings["storage:PDFExport:Location"];
var storageAccount = new CloudStorageAccount(new StorageCredentials(accountName, accountKey), true);
CloudBlobClient blobClient = storageAccount.CreateCloudBlobClient();
CloudBlobContainer container = blobClient.GetContainerReference(containerName);
// Retrieve reference to a blob named "myblob".
CloudBlockBlob blockBlob = container.GetBlockBlobReference(fileName);
blockBlob.Properties.ContentType = "application/pdf";
// Create or overwrite the "myblob" blob with contents from a local file.
using (var stream = new MemoryStream(pdf, writable: false))
{
blockBlob.UploadFromStream(stream);
}
}
return true;
}
Delete blobvar accountName = ConfigurationManager.AppSettings["storage:account:name"]; var accountKey = ConfigurationManager.AppSettings["storage:account:key"]; var containerName = ConfigurationManager.AppSettings["storage:PDFExport:Location"]; var storageAccount = new CloudStorageAccount(new StorageCredentials(accountName, accountKey), true); var deleteFolderPath = patientId+"/"+"Episode" + episodeId; CloudBlobContainer container = storageAccount.CreateCloudBlobClient().GetContainerReference(containerName); var folderDelete = container.GetDirectoryReference(deleteFolderPath); //((CloudBlob)folderDelete).DeleteIfExists();
No comments:
Post a Comment